How Developers Can Enhance Their Debugging Competencies By Gustavo Woltmann
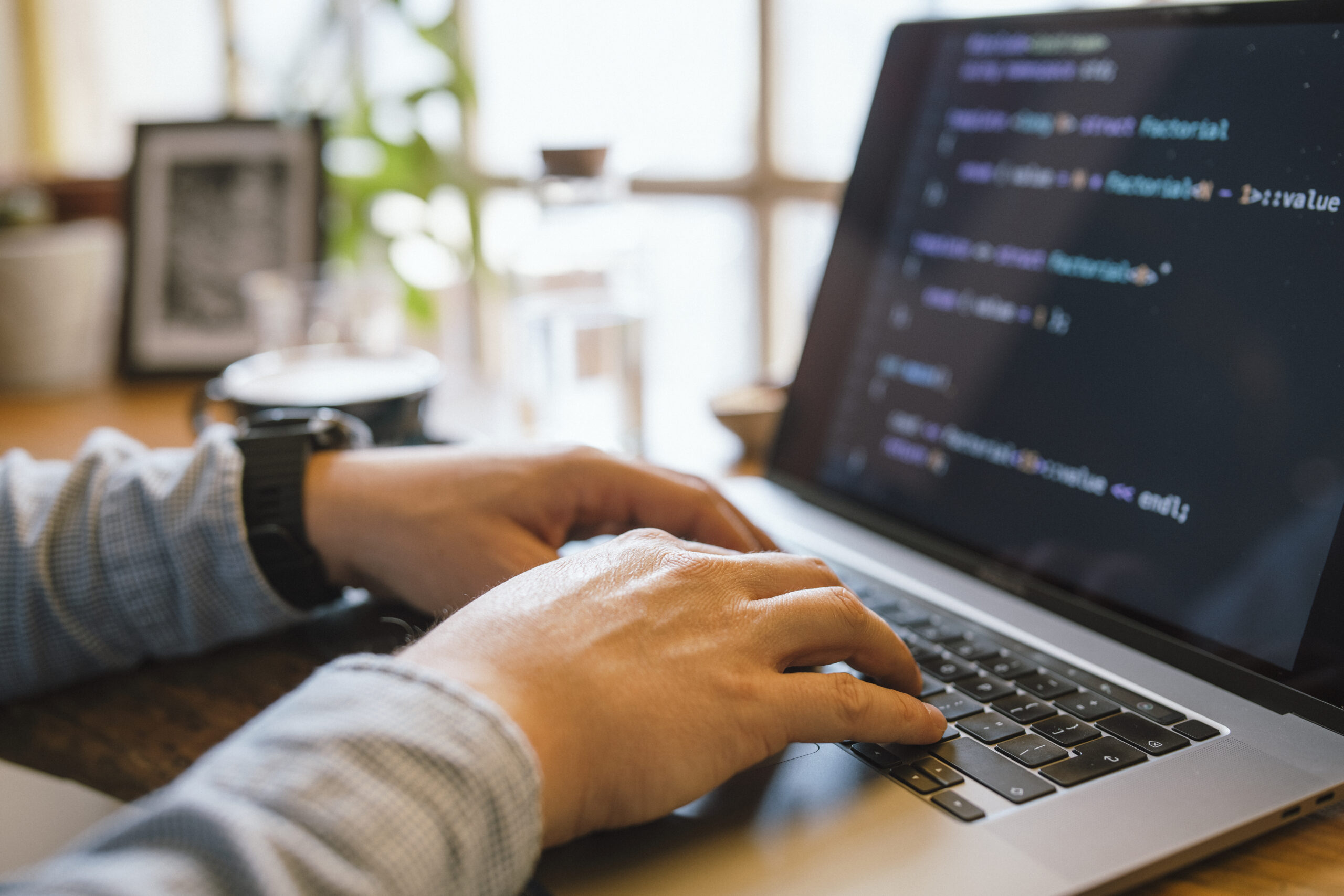
Debugging is Just about the most necessary — yet usually neglected — techniques inside of a developer’s toolkit. It's not just about fixing broken code; it’s about comprehending how and why items go Mistaken, and Mastering to Assume methodically to unravel challenges competently. Irrespective of whether you are a starter or maybe a seasoned developer, sharpening your debugging techniques can help save several hours of annoyance and considerably transform your productiveness. Allow me to share many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Instruments
One of several quickest ways builders can elevate their debugging techniques is by mastering the equipment they use daily. Whilst writing code is a person Component of growth, realizing how to connect with it properly in the course of execution is Similarly significant. Modern day improvement environments occur Outfitted with powerful debugging abilities — but several builders only scratch the surface area of what these tools can do.
Take, one example is, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, action via code line by line, and perhaps modify code about the fly. When used effectively, they let you notice exactly how your code behaves for the duration of execution, that is priceless for monitoring down elusive bugs.
Browser developer applications, for example Chrome DevTools, are indispensable for front-conclusion developers. They help you inspect the DOM, keep track of community requests, view true-time overall performance metrics, and debug JavaScript while in the browser. Mastering the console, resources, and network tabs can transform discouraging UI concerns into workable duties.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB supply deep Command more than jogging procedures and memory management. Finding out these tools might have a steeper Discovering curve but pays off when debugging efficiency challenges, memory leaks, or segmentation faults.
Over and above your IDE or debugger, become relaxed with Variation control methods like Git to be aware of code record, discover the exact second bugs ended up released, and isolate problematic changes.
Ultimately, mastering your applications means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement surroundings to ensure when troubles occur, you’re not missing in the dead of night. The higher you recognize your instruments, the greater time it is possible to commit fixing the actual issue instead of fumbling via the process.
Reproduce the Problem
One of the most significant — and infrequently forgotten — methods in powerful debugging is reproducing the trouble. Prior to jumping into your code or building guesses, developers require to produce a reliable setting or situation exactly where the bug reliably seems. With no reproducibility, fixing a bug becomes a activity of possibility, usually leading to squandered time and fragile code adjustments.
The first step in reproducing a challenge is gathering just as much context as you can. Inquire thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater detail you may have, the less difficult it results in being to isolate the exact conditions beneath which the bug occurs.
As soon as you’ve collected enough data, try to recreate the situation in your local setting. This could suggest inputting the same knowledge, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, take into consideration creating automatic checks that replicate the edge situations or point out transitions involved. These exams don't just assist expose the challenge but will also stop regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would transpire only on sure operating techniques, browsers, or underneath individual configurations. Using resources like virtual devices, containerization (e.g., Docker), or cross-browser testing platforms might be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a attitude. It calls for endurance, observation, in addition to a methodical solution. But once you can regularly recreate the bug, you are presently halfway to fixing it. Using a reproducible situation, You need to use your debugging equipment far more proficiently, take a look at probable fixes safely and securely, and converse far more Plainly using your crew or end users. It turns an abstract complaint right into a concrete obstacle — Which’s the place developers prosper.
Go through and Realize the Error Messages
Mistake messages will often be the most beneficial clues a developer has when a thing goes Erroneous. As an alternative to viewing them as irritating interruptions, builders really should understand to deal with error messages as immediate communications through the program. They frequently show you just what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Start out by looking through the message diligently and in full. Quite a few developers, specially when beneath time stress, look at the initial line and immediately start out producing assumptions. But further while in the mistake stack or logs may possibly lie the accurate root bring about. Don’t just copy and paste mistake messages into engines like google — study and fully grasp them initial.
Crack the error down into sections. Is it a syntax error, a runtime exception, or simply a logic error? Will it stage to a certain file and line number? What module or operate brought on it? These queries can guideline your investigation and level you towards the responsible code.
It’s also valuable to understand the terminology in the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java typically abide by predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging process.
Some mistakes are obscure or generic, As well as in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify connected log entries, enter values, and up to date changes inside the codebase.
Don’t forget about compiler or linter warnings possibly. These normally precede bigger concerns and supply hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, decrease debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most highly effective instruments in a very developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, serving to you fully grasp what’s going on underneath the get more info hood while not having to pause execution or phase throughout the code line by line.
A superb logging method begins with realizing what to log and at what degree. Common logging levels include DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through progress, Details for standard activities (like effective start-ups), Alert for likely concerns that don’t break the applying, ERROR for real problems, and Lethal once the system can’t go on.
Prevent flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Target important situations, condition modifications, enter/output values, and demanding conclusion details within your code.
Structure your log messages Obviously and consistently. Include things like context, like timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed methods or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs Allow you to observe how variables evolve, what circumstances are fulfilled, and what branches of logic are executed—all with out halting This system. They’re especially worthwhile in production environments the place stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Eventually, clever logging is about balance and clarity. By using a perfectly-believed-out logging technique, you could reduce the time it requires to identify issues, obtain further visibility into your purposes, and improve the General maintainability and dependability of your respective code.
Think Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To efficiently establish and take care of bugs, developers should strategy the method just like a detective resolving a secret. This mentality helps break down complicated concerns into manageable areas and observe clues logically to uncover the foundation induce.
Get started by accumulating proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance problems. Much like a detective surveys a crime scene, gather as much related info as you are able to with out jumping to conclusions. Use logs, test cases, and person experiences to piece jointly a transparent image of what’s taking place.
Subsequent, form hypotheses. Ask you: What can be creating this behavior? Have any variations not long ago been designed on the codebase? Has this concern occurred right before underneath related situations? The goal should be to slim down prospects and determine opportunity culprits.
Then, take a look at your theories systematically. Make an effort to recreate the issue inside of a managed atmosphere. If you suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the results direct you closer to the reality.
Spend shut focus to compact information. Bugs often cover within the the very least expected destinations—like a lacking semicolon, an off-by-1 mistake, or a race issue. Be thorough and individual, resisting the urge to patch the issue with no absolutely comprehension it. Non permanent fixes could disguise the real dilemma, just for it to resurface afterwards.
Finally, continue to keep notes on Everything you tried using and discovered. Equally as detectives log their investigations, documenting your debugging system can conserve time for long run issues and support Many others comprehend your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in complicated programs.
Produce Checks
Writing exams is one of the best solutions to help your debugging abilities and All round growth effectiveness. Checks don't just help catch bugs early but additionally serve as a safety Internet that provides you self confidence when building variations for your codebase. A nicely-examined application is simpler to debug mainly because it helps you to pinpoint exactly where and when a problem occurs.
Start with device checks, which focus on individual functions or modules. These small, isolated checks can immediately expose irrespective of whether a specific bit of logic is Doing the job as envisioned. When a test fails, you immediately know where to look, considerably reducing some time expended debugging. Device exams are Particularly useful for catching regression bugs—issues that reappear after Beforehand currently being mounted.
Up coming, integrate integration checks and conclusion-to-conclude tests into your workflow. These help ensure that several areas of your application do the job jointly efficiently. They’re especially practical for catching bugs that come about in intricate methods with multiple parts or providers interacting. If something breaks, your assessments can let you know which part of the pipeline failed and under what ailments.
Composing checks also forces you to Believe critically regarding your code. To test a element correctly, you need to grasp its inputs, expected outputs, and edge scenarios. This degree of being familiar with By natural means potential customers to higher code composition and fewer bugs.
When debugging a concern, writing a failing take a look at that reproduces the bug may be a strong first step. After the take a look at fails regularly, it is possible to focus on repairing the bug and check out your check move when The difficulty is resolved. This technique makes certain that the identical bug doesn’t return Sooner or later.
To put it briefly, creating assessments turns debugging from the irritating guessing match right into a structured and predictable process—aiding you capture extra bugs, quicker and a lot more reliably.
Choose Breaks
When debugging a tricky concern, it’s effortless to be immersed in the problem—looking at your display for hrs, hoping Alternative following Answer. But The most underrated debugging instruments is solely stepping absent. Having breaks aids you reset your brain, lessen stress, and infrequently see The difficulty from the new standpoint.
If you're as well close to the code for as well extended, cognitive fatigue sets in. You could commence overlooking apparent problems or misreading code that you just wrote just hrs earlier. Within this state, your Mind results in being fewer economical at problem-resolving. A brief stroll, a coffee break, and even switching to a special job for ten–quarter-hour can refresh your emphasis. Several developers report getting the foundation of a difficulty after they've taken the perfect time to disconnect, permitting their subconscious operate within the background.
Breaks also enable avert burnout, Specifically throughout for a longer period debugging periods. Sitting before a monitor, mentally caught, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you just before.
If you’re caught, a good guideline would be to established a timer—debug actively for 45–60 minutes, then have a 5–ten minute split. Use that time to maneuver about, extend, or do some thing unrelated to code. It could really feel counterintuitive, Primarily below limited deadlines, nevertheless it basically results in a lot quicker and simpler debugging in the long run.
In a nutshell, having breaks isn't an indication of weak spot—it’s a smart approach. It presents your brain Place to breathe, increases your viewpoint, and will help you steer clear of the tunnel vision That usually blocks your development. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Every Bug
Every single bug you come upon is more than just A brief setback—It is really an opportunity to expand for a developer. Whether it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can teach you some thing worthwhile when you take the time to reflect and evaluate what went Mistaken.
Start out by inquiring you a few important queries after the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code testimonials, or logging? The solutions typically expose blind spots with your workflow or comprehension and allow you to Make more robust coding behaviors shifting forward.
Documenting bugs can also be an excellent habit. Continue to keep a developer journal or manage a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—that you could proactively steer clear of.
In workforce environments, sharing That which you've figured out from a bug with your friends might be Specifically potent. Whether it’s via a Slack message, a brief publish-up, or a quick awareness-sharing session, serving to Other individuals avoid the similar concern boosts team performance and cultivates a stronger Discovering tradition.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll begin appreciating them as necessary elements of your enhancement journey. In any case, some of the ideal builders will not be those who compose fantastic code, but people who consistently find out from their issues.
Ultimately, Just about every bug you repair provides a completely new layer in your talent set. So up coming time you squash a bug, have a instant to reflect—you’ll appear absent a smarter, much more capable developer thanks to it.
Conclusion
Strengthening your debugging competencies will take time, exercise, and tolerance — nevertheless the payoff is big. It will make you a more effective, self-confident, and able developer. Another time you're knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.